Web3 Ethereum DAPP
Try It! Visit RepoTechnologies/Software used: Solidity, React, Hardhat, Alchemy, and Vite
- Personal Project
- Smart contract created with Solidity and deployed with Alchemy and Hardhat
- Gets user wallet information through browser
- Sends blockchain transactions using Ropsten Ethereum
- Utilizes Giphy API to find gifs for a transaction with a given keyword
This project was a ton of fun and was created using a youtube tutorial. I learned so much about how the blockchain functions and how to interact with a smart contract from my front-end. A major problem I ran into right at the end of my project is that I made a typo in my smart contract which caused my receiver address to be undefined, and I had already deployed it by the time I figured that out. This was a major issue because the smart contract acts as a backend server for decentralized applications and once its deployed its immutable meaning you can't simply re-upload your code. In order to fix any issues with a smart contract you have to re-upload your smart contract entirely and re-link the contract address and new ABI. Through fixing this error I also learned that its very expensive to make mistakes by having to deploy contracts again and luckily I used a test network with Ropsten ETH, otherwise i'd be spending $500+ dollars in mainnet ETHER if I chose to deploy my app on the main network.
Solidity Smart Contract
//SPDX-License-Identifier: UNLICENSED
pragma solidity ^0.8.0;
contract Transactions {
uint256 transactionCount;
event Transfer(address from, address receiver, uint amount, string message, uint256 timestamp, string keyword);
struct TransferStruct {
//properties of the object
address sender;
address receiver;
uint amount;
string message;
uint256 timestamp;
string keyword;
}
TransferStruct[] transactions;
function addToBlockchain(address payable receiver, uint amount, string memory message, string memory keyword) public{
transactionCount += 1;
//only adds our transaction to the array, doesn't actually make the transaction on the blockchain
transactions.push(TransferStruct(msg.sender, receiver, amount, message, block.timestamp, keyword));
//executes the transaction on the blockchain
emit Transfer(msg.sender, receiver, amount, message, block.timestamp, keyword);
}
function getAllTransactions() public view returns (TransferStruct[] memory){
return transactions;
}
function getTransactionCount() public view returns (uint256){
return transactionCount;
}
}
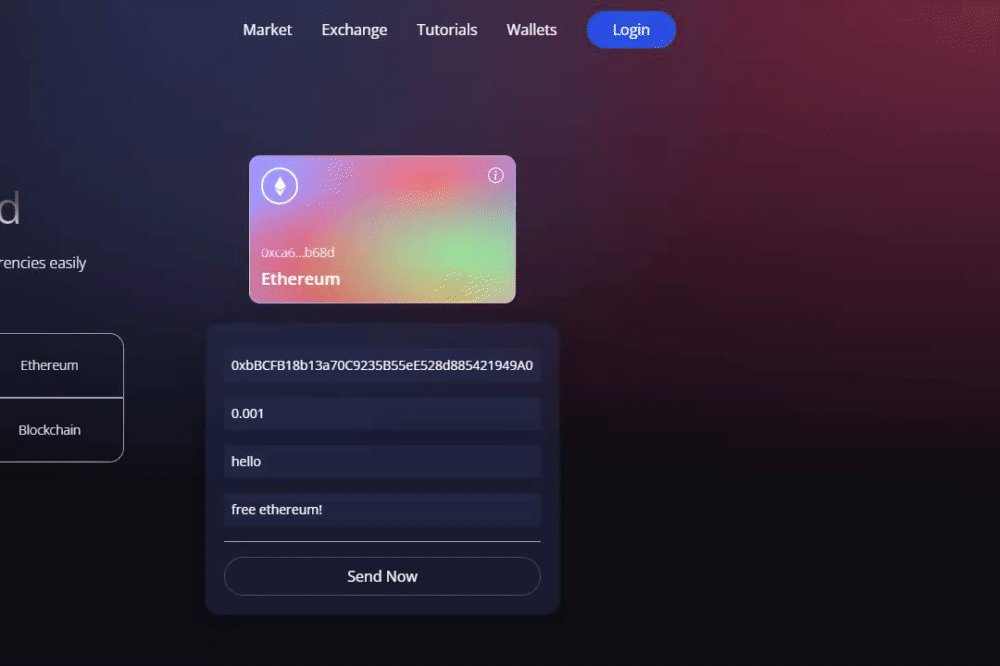